Unity 3D Game Engine – Android – Accelerometer – RAW Data – Translate and Object
Inside Hierarchy create:
– Main Camera
– GUI Text X
– GUI Text Y
– GUI Text Z
– Cube (Game Object), attach ‘AccelerometerTest.js’
AccelerometerTest.js
#pragma strict
// Hierarchy DRAG E DROP over var GUI Text in Inspector
var scoreTextX : GUIText;
var scoreTextY : GUIText;
var scoreTextZ : GUIText;
function Update () {
var dir : Vector3 = Vector3.zero; // Shorthand for writing Vector3(0, 0, 0)
dir.x = Input.acceleration.x;
dir.y = Input.acceleration.y;
dir.z = Input.acceleration.z;
scoreTextX.text = "acceleration.x: " + dir.x;
scoreTextY.text = "acceleration.y: " + dir.y;
scoreTextZ.text = "acceleration.z: " + dir.z;
}
Hierarchy> Cube> Inspector> AccelerometerTest.js assign GUI Text to public var scoreText
Here the final result:
dir.x = Input.acceleration.x; from 1 to -1 -> example: 0.1234567 or -0.1234567
dir.y = Input.acceleration.y; from 1 to -1
dir.z = Input.acceleration.z; from 1 to -1

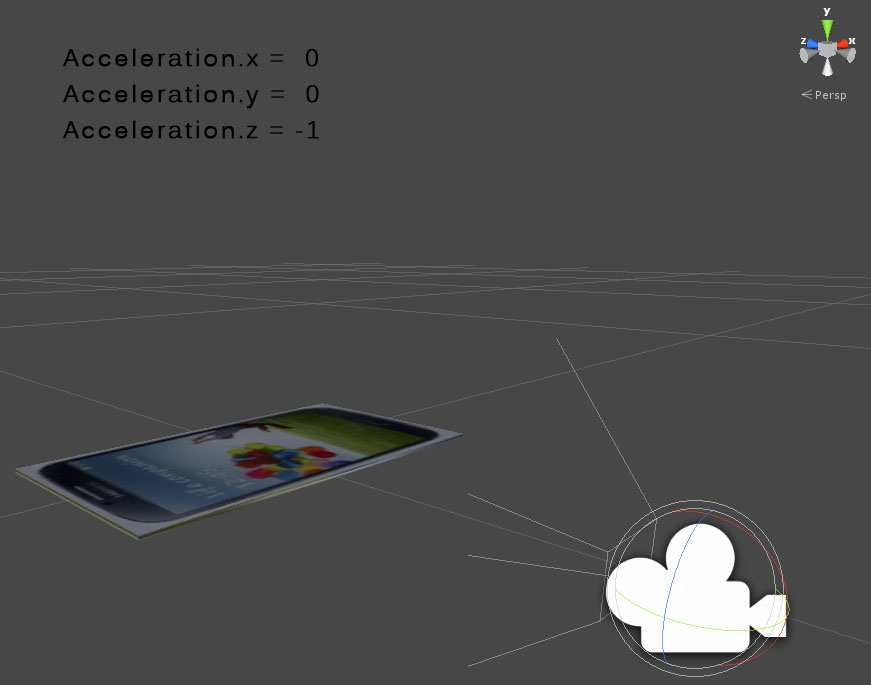
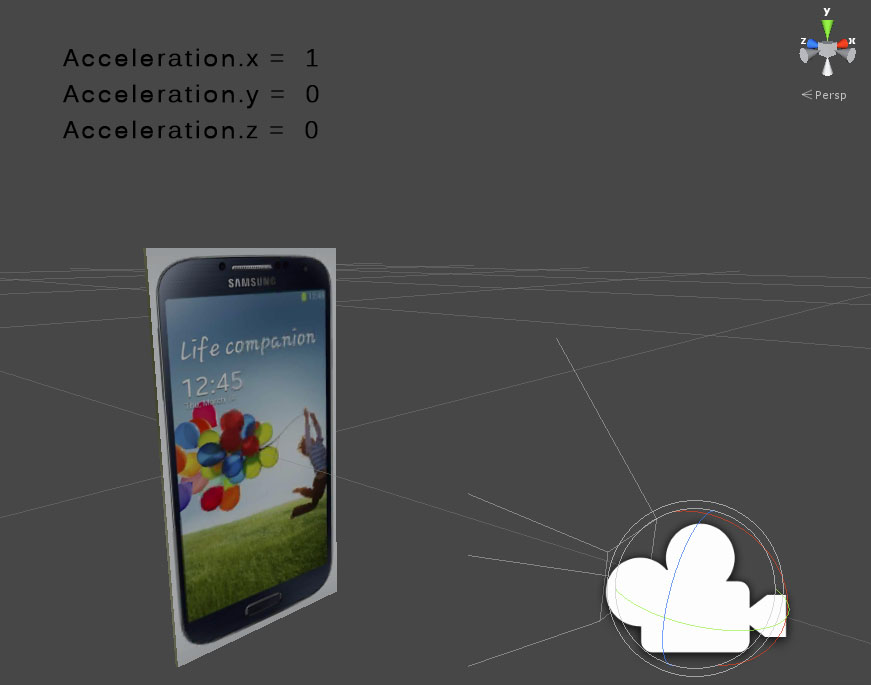
To translate the Cube using Accelerometer data (basic):
AccelerometerTest.js
#pragma strict
function Update ()
{
transform.Translate(Input.acceleration.x, 0, -Input.acceleration.z);
}
To translate the Cube using Accelerometer data (advanced):
AccelerometerTest.js
#pragma strict
// Move object using accelerometer
var speed = 10.0;
// Hierarchy DRAG E DROP over var GUI Text in Inspector
var scoreTextX : GUIText;
function Update () {
var dir : Vector3 = Vector3.zero; // Shorthand for writing Vector3(0, 0, 0)
dir.x = Input.acceleration.x;
scoreTextX.text = "acceleration.x: " + dir.x;
// clamp acceleration vector to unit sphere
if (dir.sqrMagnitude > 1)
dir.Normalize();
// Make it move 10 meters per second instead of 10 meters per frame...
dir *= Time.deltaTime;
// Move object
transform.Translate (dir * speed);
}
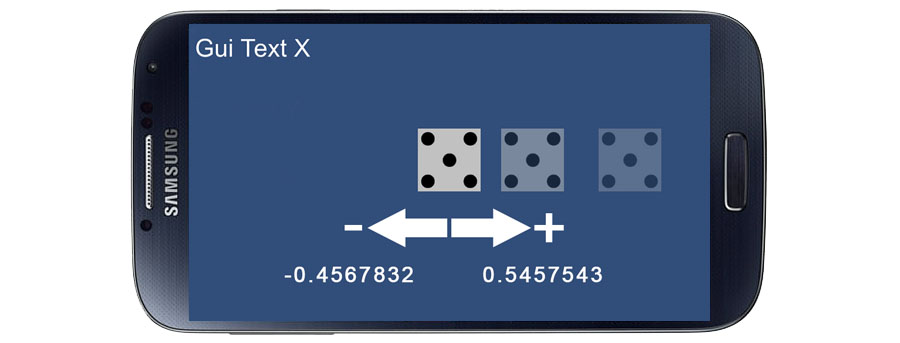