PHP – MySQL – Simple Calendar
DOWNLOAD
We want a calendar like this:
—–
Title of the event
Start date – End date
Content bla bla bla … Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Aenean commodo ligula eget dolor. Aenean massa. Cum sociis natoque penatibus et magnis dis parturient montes, nascetur ridiculus mus. Donec quam felis, ultricies nec, pellentesque eu, pretium quis, sem. Nulla consequat massa quis enim. Donec pede justo, fringilla vel, aliquet nec, vulputate eget, arcu. In enim justo, rhoncus ut, imperdiet a, venenatis vitae, justo. Nullam dictum felis eu pede mollis pretium. Integer tincidunt. Cras dapibus. Vivamus elementum semper nisi. Aenean vulputate eleifend tellus.
Link>
—–
Create database
Entriamo in phpMyAdmin
In alto posizioniamoci su localhost> mydatabase
Usando phpMyAdmin creiamo:
Colonna di sinistra> ‘Crea tabella’> MyISAM
Nome tabella: calendar’
Struttura> aggiungere i campi
Campo: id
Tipo: INT
Lunghezza: 20
Predefinito: Nessuno
Null: deselezionato
Indice: PRIMARY
AUTO_INCREMENT: selezionato
Campo: title
Tipo: VARCHAR
Lunghezza: 255
Predefinito: Nessuno
Null: deselezionato
Indice: nessuno
AUTO_INCREMENT: deselezionato
Campo: link
Tipo: VARCHAR
Lunghezza: 255
Predefinito: Nessuno
Null: deselezionato
Indice: nessuno
AUTO_INCREMENT: deselezionato
Campo: content
Tipo: LONGTEXT -> it must contain max 4,294,967,295 characters
Lunghezza: -> non specifichiamo nulla
Predefinito: Nessuno
Null: deselezionato
Indice: nessuno
AUTO_INCREMENT: deselezionato
Campo: startDate
Tipo: DATETIME -> Format: YYYY-MM-DD HH:MM:SS Example: 2014-12-31 23:59:59
Campo: endDate
Tipo: DATETIME -> Format: YYYY-MM-DD HH:MM:SS Example: 2014-12-31 23:59:59
PhpMyAdmin
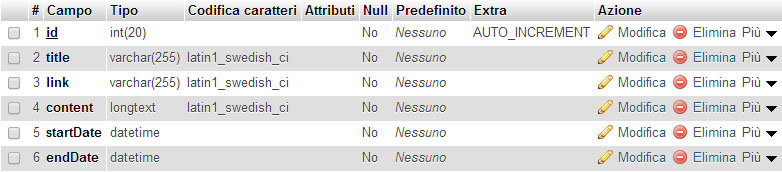
calendar_input_form.php
The input form
<!-- CONNESSIONE DB START -->
<?php
// Create DATABASE connection - START
// Statement: mysqli_connect(host,username,password,dbname)
// NOTICE: se lo script è installato nello stesso server del Data Base, host->localhost
$con=mysqli_connect("localhost","lucedigi_user","mypassword","lucedigi_testphp");
// Check connection
if (mysqli_connect_errno())
{
echo "<br> Failed to connect to MySQL: " . mysqli_connect_error();
}
else
{
echo "Great! Connect to MySQL!<br><br>";
}
// Create DATABASE connection - END
?>
<!-- CONNESSIONE DB END -->
<html>
<body>
<!-- FORM INSERT START -->
<!-- Send data to calendar_insert_engine.php -->
<form action="calendar_insert_engine.php?action=addEvent" method="post">
Title: <input type="text" name="title"><br/>
Link: <input type="text" name="link"><br/>
Start date (YYYY-MM-DD HH:MM): <input type="text" name="startDate"><br/>
End date (YYYY-MM-DD HH:MM): <input type="text" name="endDate"><br/>
Content: <br/>
<textarea rows="15" cols="30" name="content"></textarea><br/>
<input type="submit" value="Insert Event">
</form>
<!-- FORM INSERT END -->
</body>
</html>
<!-- QUERY DB START -->
<?php
// SELECT asterisco (tutti i dati) dalla tabella START
// inserisco i dati nella variabile $result
// ordinati in base a startDate in ordine decrescente
$result = mysqli_query($con,"SELECT * FROM calendar ORDER BY startDate DESC");
echo "<strong>Calendar - Event List: (Order by startDate DESC)</strong>";
echo "<br><br>ID - Title - Link - Start date - End date - Content<br>";
// Restituisce il set di record come un array
// ad ogni chiamata viene restituita la riga successiva
while($row = mysqli_fetch_array($result))
{
// Visualizza a video i dati
echo $row['id'] . " - " .$row['title'] . " - " . $row['link'] . " - " . $row['startDate'] . " - " . $row['endDate'] . " - " . $row['content'];
echo "<br>";
}
// SELECT asterisco (tutti i dati) dalla tabella END
mysqli_close($con);
echo "<br>Great! Connection Closed!";
?>
<!-- QUERY DB END -->
calendar_insert_engine.php
The PHP engine to store data
<?php
$host="localhost"; //lasciare com'è se utilizzate bluehost
$username="lucedigi_user";
$password="mypassword";
$db_name="lucedigi_testphp"; // database name
$tbl_name="calendar"; //Indicate la tabella presente nel database a cui si deve collegare
// Connetti al server e seleziona il database
mysql_connect("$host", "$username", "$password")or die("cannot connect");
mysql_select_db("$db_name")or die("DB non connesso");
// Ottengo i dati dal form HTML
// Se dal form arriva - action=addEvent - esegui il seguente
if($_GET['action'] == 'addEvent'){
$title = mysql_real_escape_string($_POST['title']);
$link = mysql_real_escape_string($_POST['link']);
$content = mysql_real_escape_string($_POST['content']);
$startDate = date('Y-m-d H:i:s', strtotime($_POST['startDate'] . ":00"));
$endDate = date('Y-m-d H:i:s', strtotime($_POST['endDate'] . ":00"));
// Invio una query per inserire i dati
mysql_query("INSERT INTO calendar VALUES (null, '$title', '$link', '$content', '$startDate', '$endDate');");
echo "Great! New Record Inserted!";
}
?>
IMPROVE INPUT FORM WITH date tag
<!-- CONNESSIONE DB START -->
<?php
// Create DATABASE connection - START
// Statement: mysqli_connect(host,username,password,dbname)
// NOTICE: se lo script è installato nello stesso server del Data Base, host->localhost
$con=mysqli_connect("localhost","lucedigi_user","mypassword","lucedigi_testphp");
// Check connection
if (mysqli_connect_errno())
{
echo "<br> Failed to connect to MySQL: " . mysqli_connect_error();
}
else
{
echo "Great! Connect to MySQL!<br><br>";
}
// Create DATABASE connection - END
?>
<!-- CONNESSIONE DB END -->
<html>
<body>
<!-- FORM INSERT START -->
<!-- Send data to calendar_insert_engine.php -->
<form action="calendar_insert_engine.php?action=addEvent" method="post">
Title: <input type="text" name="title"><br/>
Link: <input type="text" name="link"><br/>
Start date (this send -> 2018-02-05): <input type="date" name="startDate"><br/>
End date (this send -> 2018-02-05): <input type="date" name="endDate"><br/>
Content: <br/>
<textarea rows="15" cols="30" name="content"></textarea><br/>
<input type="submit" value="Insert Event">
</form>
<!-- FORM INSERT END -->
</body>
</html>
<!-- QUERY DB START -->
<?php
// SELECT asterisco (tutti i dati) dalla tabella START
// inserisco i dati nella variabile $result
// ordinati in base a startDate in ordine decrescente
$result = mysqli_query($con,"SELECT * FROM calendar ORDER BY startDate DESC");
echo "<strong>Calendar - Event List: (Order by startDate DESC)</strong>";
echo "<br><br>ID - Title - Link - Start date - End date - Content<br>";
// Restituisce il set di record come un array
// ad ogni chiamata viene restituita la riga successiva
while($row = mysqli_fetch_array($result))
{
// Visualizza a video i dati
echo $row['id'] . " - " .$row['title'] . " - " . $row['link'] . " - " . $row['startDate'] . " - " . $row['endDate'] . " - " . $row['content'];
echo "<br>";
}
// SELECT asterisco (tutti i dati) dalla tabella END
mysqli_close($con);
echo "<br>Great! Connection Closed!";
?>
<!-- QUERY DB END -->
<?php
$host="localhost"; //lasciare com'è se utilizzate bluehost
$username="lucedigi_user";
$password="mypassword";
$db_name="lucedigi_testphp"; // database name
$tbl_name="calendar"; //Indicate la tabella presente nel database a cui si deve collegare
// Connetti al server e seleziona il database
mysql_connect("$host", "$username", "$password")or die("cannot connect");
mysql_select_db("$db_name")or die("DB non connesso");
// Ottengo i dati dal form HTML
// Se dal form arriva - action=addEvent - esegui il seguente
if($_GET['action'] == 'addEvent'){
$title = mysql_real_escape_string($_POST['title']);
$link = mysql_real_escape_string($_POST['link']);
$content = mysql_real_escape_string($_POST['content']);
$startDate = date('Y-m-d H:i:s', strtotime($_POST['startDate'] . " 00:00:00"));
$endDate = date('Y-m-d H:i:s', strtotime($_POST['endDate'] . " 00:00:00"));
// Invio una query per inserire i dati
mysql_query("INSERT INTO calendar VALUES (null, '$title', '$link', '$content', '$startDate', '$endDate');");
echo "Great! New Record Inserted!";
}
?>
Notice:
the HTML form send data as 2018-02-05
the PHP script add ” 00:00:00″ -> strtotime($_POST[‘startDate’] . ” 00:00:00″
because MySQL DATETIME needs the format: YYYY-MM-DD HH:MM:SS Example: 2014-12-31 23:59:59